Especially in the cloud era, flexible connectivity is becoming increasingly important. Cloud systems are to be integrated more closely with its on-premise counterparts. For this purpose, most cloud providers offer sophisticated interfaces, which are usually realized as so-called REST APIs.
In this article, I'll show you how to connect SalesForce and SAP BW with ABAP and explore the integration options. For reading and writing large amounts of data, Salesforce provides the Bulk API 2.0 interface.
When does a direct connection to a cloud system make sense?
A direct connection to Salesforce makes sense if you want to control and adapt the data loading process from the SAP system. Another use case arises if you want a strong integration between SAP system and Salesforce. You also need a direct connection if there is no middleware through which the communication could take place.
Is such connection secure?
In such a scenario, SAP BW server acts as an HTTP client and establishes a connection to Salesforce. To do this, you must configure the firewall appropriately and allow an outbound connection to the IP addresses of Salesforce. Incoming connections to the SAP BW server are not possible, which minimizes the risk.
Integration in SAP BW
The connection to Salesforce can be integrated into existing loads using process chains. You can find scenarios for read and write access below.
Read Access:
Process chain for reading data from Salesforce |
|
Step 1 |
Execute a program that reads data from Salesforce and writes it request-based to an ADSO. Any errors that occur during reading can be written to the process chain log |
Step 2 |
Activate ADSO |
Write access:
Process chain for writing data in Salesforce |
|
Step 1 |
Load ADSO with data to be transferred to Salesforce |
Step 2 |
Run a program that sends data to Salesforce. Any errors that occur during transmission can be written to the process chain log. |
Salesforce Bulk API 2.0 interface in detail
Let's take a closer look at the Salesforce interface Bulk API 2.0. This API is specially designed for large amounts of data and allows read and write access. This way you can put different queries on objects in Salesforce or flexibly change data in Salesforce via INSERT / UPDATE / DELETE commands. You can view the details and current documentation on the Salesforce page.
SAP BW, HANA Native and SAP Datawarehouse Cloud - Download the comparison
Encapsulated logic for easy connection
For easier integration of new tables, it's a good idea to encapsulate the Salesforce API-specific logic on the SAP side. Thus, no specific know-how about the functionality of the interface is needed when you add new connections.
A read access call could be implemented in an ABAP class as follows:
* Step 1: Login and important parameters for BULK API 2.0
DATA lo_sf TYPE REF TO zcl_sf_bulk_api_2_0.
lo_sf = zcl_sf_bulk_api_2_0=>login_by_url(
i_host_login = 'test.salesforce.com'
i_path_login = '/services/oauth2/token'
i_bulk_api_2_0_vers = 'v47.0'
i_https = 'X'
i_port = '443'
i_clientid = 'XXX'
i_secret = 'XXX'
i_user = 'XXX'
i_pw = 'XXX'
).
* Step 2: Read access to Salesforce
CALL METHOD lo_sf->query_data
EXPORTING
i_sql = | SELECT Id, Name FROM Account LIMIT 100|
IMPORTING
e_result = DATA(result)
e_job_state = DATA(l_job_state)
.
* E_Result contains Salesforce data for further processing
Logic for a Read Access from ABAP explained step by step
Use SAP Server as HTTP Client
To execute the connection from the SAP side, the SAP server must call the Salesforce interface as an HTTP client. For this, SAP provides the class cl_http_client. The detailed documentation of this class can be found here.
oAuth 2.0 Login
To log in to Salesforce, certain information must be passed in the header and form fields. Details about the login process can be found on the Salesforce page.
To implement the login process with the ABAP class, it is adapted as follows:
i_client->request->set_header_field(
EXPORTING
name = 'Content-Type' " Name of the header field
value = 'application/x-www-form-urlencoded' " Value of the header field
).
i_client->request->set_form_field(
EXPORTING
name = 'grant_type' " Name of the form field
value = 'password' " Value of the form field
).
i_client->request->set_form_field(
EXPORTING
name = 'client_id' " Name of the form field
value = i_clientid " Value of the form field
).
i_client->request->set_form_field(
EXPORTING
name = 'client_secret' " Name of the form field
value = i_secret " Value of the form field
).
i_client->request->set_form_field(
EXPORTING
name = 'username' " Name of the form field
value = i_user " Value of the form field
).
i_client->request->set_form_field(
EXPORTING
name = 'password' " Name of the form field
value = i_pw " Value of the form field
).
i_client->request->set_method(
method = 'POST'
).
From the response of the server, the access token can be taken, which must be carried along in the header with all further accesses to the Salesforce API. In addition, you will receive the instance, to which the logged in user has access. This instance will be used for all other requests.
Send job ID and query
After you have received the access token and instance, you can use it with any further queries. Here you must adjust the body and header as follows:
* for example DATA(sql) = | SELECT Id, Name FROM Account LIMIT 100|.
l_body_job =
|\{| &&
|"operation" : "query",| &&
|"query": "{ sql }"| &&
|\}|.
*get JobID and send query
DATA(l_uri_job) = |/services/data/{ mv_bulk_api_2_0_vers }/jobs/query/|.
* set request uri (/<path>[?<querystring>])
cl_http_utility=>set_request_uri( request = lo_client->request
uri =l_uri ).
IF i_method IS NOT INITIAL.
lo_client->request->set_method(
method = ‘POST’
).
ENDIF.
lo_client->request->set_header_field(
EXPORTING
name = 'Accept' " Name of the header field
value = 'application/json' " Value of the header field
).
lo_client->request->set_header_field(
EXPORTING
name = 'Authorization' " Name of the header field
value = |Bearer { mv_sessionid }| " Access Token
).
IF i_body IS NOT INITIAL.
* convert body to xstring as this is better consumed by salesforce avoiding errors in Update requests.
DATA lx_body TYPE xstring.
CALL FUNCTION 'SCMS_STRING_TO_XSTRING'
EXPORTING
text = i_body " variable type string
IMPORTING
buffer = lx_body. " variable type xstring
lo_client->request->set_data( lx_body ).
ENDIF.
Check the status of the query
In the last step, we started a query to the Salesforce system. Depending on the amount of data, it may take a few seconds to minutes for the query to be processed and for the result to be available for download.
To check the current status of your request, you can send a status query to Salesforce. In response, you will get back the current state. You should periodically check if the job has been completed correctly and completely. The JobComplete state corresponds to a successfully completed query. You can find a description how to structure your status query here.
Retrieve results
After getting back a JobComplete state, you can retrieve the results via a GET request. To do this, a new query with the following path and header is sent to Salesforce.
DATA(l_uri_queryresults) = |/services/data/{ mv_bulk_api_2_0_vers }/jobs/query/{ mv_job_id }/results/|.
IF i_method IS NOT INITIAL.
lo_client->request->set_method(
method = i_method
).
ENDIF.
lo_client->request->set_header_field(
EXPORTING
name = 'Accept' " Name of the header field
value = 'application/json' " Value of the header field
).
lo_client->request->set_content_type( content_type = ‘GET’).
lo_client->request->set_header_field(
EXPORTING
name = 'Authorization' " Name of the header field
value = |Bearer { mv_sessionid }| " Value of the header field
).
Summary
It is possible to connect a cloud system to SAP BW with a manageable effort. This connection offers countless integration options that can be exploited with this or a similar approach.
If you are looking for an integration with another cloud provider or interface, please take a look at our web connectivity offer:
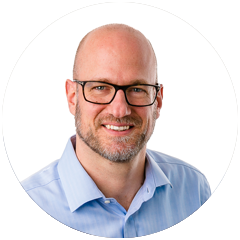